Over the past year, my PowerShell scripting has evolved tremendously. One of the biggest game‑changers has been GitHub Copilot. In this post, I’ll share how I’ve integrated Copilot into my daily PowerShell workflow—and why its latest features, including the enhanced Copilot Edits for multi‑file modifications and code review, are taking productivity to a whole new level.
Why GitHub Copilot for PowerShell?
If you’ve ever spent too long wrestling with repetitive code or hunting down the right syntax, you know that even seasoned PowerShell users can benefit from a little extra help. GitHub Copilot leverages AI to provide intelligent code completions, generate snippets from natural language comments, and even propose coordinated changes across multiple files. The result? Faster script development, fewer errors, and more time to focus on the core logic instead of boilerplate.
Getting Started: Setting Up Copilot
The first step is to install the GitHub Copilot extension in your favorite IDE. I use Visual Studio Code for most of my PowerShell development. Once installed, simply sign in with your GitHub account (whether you’re on a free trial or a paid plan, you’re all set). Here’s a quick checklist:
- Install VS Code (or your preferred editor that supports Copilot).
- Install the GitHub Copilot Extension from the marketplace.
- Sign In to GitHub and enable the extension.
- Open a PowerShell file and start typing a comment describing your intent (for example,
# Get all running services
).
Within seconds, Copilot suggests code that you can accept (using Tab) or modify further. It’s like having a pair‑programmer always by your side.
Streamlining Your Workflow with Copilot Auto‑Completion
One of the easiest ways to harness GitHub Copilot is through inline auto-completion in your editor. As you type a comment describing your task, Copilot instantly suggests a snippet that you can accept, reject, or refine. This makes it perfect for quick wins and small bits of logic you want to insert into your script.
Example: Inline in the Editor
Suppose you want to list all running services in PowerShell, displaying their start type, status, and display name. You also want to catch any errors that occur. In your .ps1
file, type a natural-language comment like this:
# List all running services and display their start type, status, and display name.
# Use the Get-Service cmdlet to retrieve the services, then use Format-Table to display
# the results in a table format. Use proper error handling to catch any exceptions.
Almost immediately, Copilot suggests a code block similar to:
try {
$services = Get-Service | Where-Object { $_.Status -eq 'Running' }
$services | Format-Table Name, Status, StartType -AutoSize
} catch {
Write-Host "An error occurred: $($_.Exception.Message)"
}
You can accept this inline suggestion by pressing Tab (or your configured accept‑completion key), then tweak it further as needed. This inline approach keeps you in the flow and helps you quickly produce a working snippet.
Taking It Further: GitHub Copilot Edits
One of the most exciting recent updates is GitHub Copilot Edits. This feature goes beyond single‑file completions by allowing multi‑file editing and a more conversational, iterative approach to code changes.
What Are Copilot Edits?
Copilot Edits lets you start an AI‑powered editing session directly from your IDE. You can:
- Apply changes across multiple files at once.
- Review inline diffs of the suggested edits.
- Use either Edit Mode (where you control the working set) or Agent Mode (where Copilot autonomously determines what needs to be changed).
If you want to apply or modify code across multiple files—or if you prefer a more conversational workflow—Copilot Edits offers an expanded approach. Here’s how you could handle the same scenario (listing running services with error handling) using Copilot Edits:
- Add Your Files to the Working Set
- In VS Code, open the Copilot Edits view (typically on the right).
- Click Add Files… and select the
.ps1
file(s) where you want the changes applied.
- Enter Your Prompt
- In the Copilot Edits panel, type something like:
List all running services and display their start type, status, and display name. Use the Get-Service cmdlet and Format-Table. Include proper error handling.
- Copilot then analyzes your code and suggests changes or additions.
- In the Copilot Edits panel, type something like:
- Review Suggested Edits
- Copilot displays a diff showing what lines it wants to add or modify.
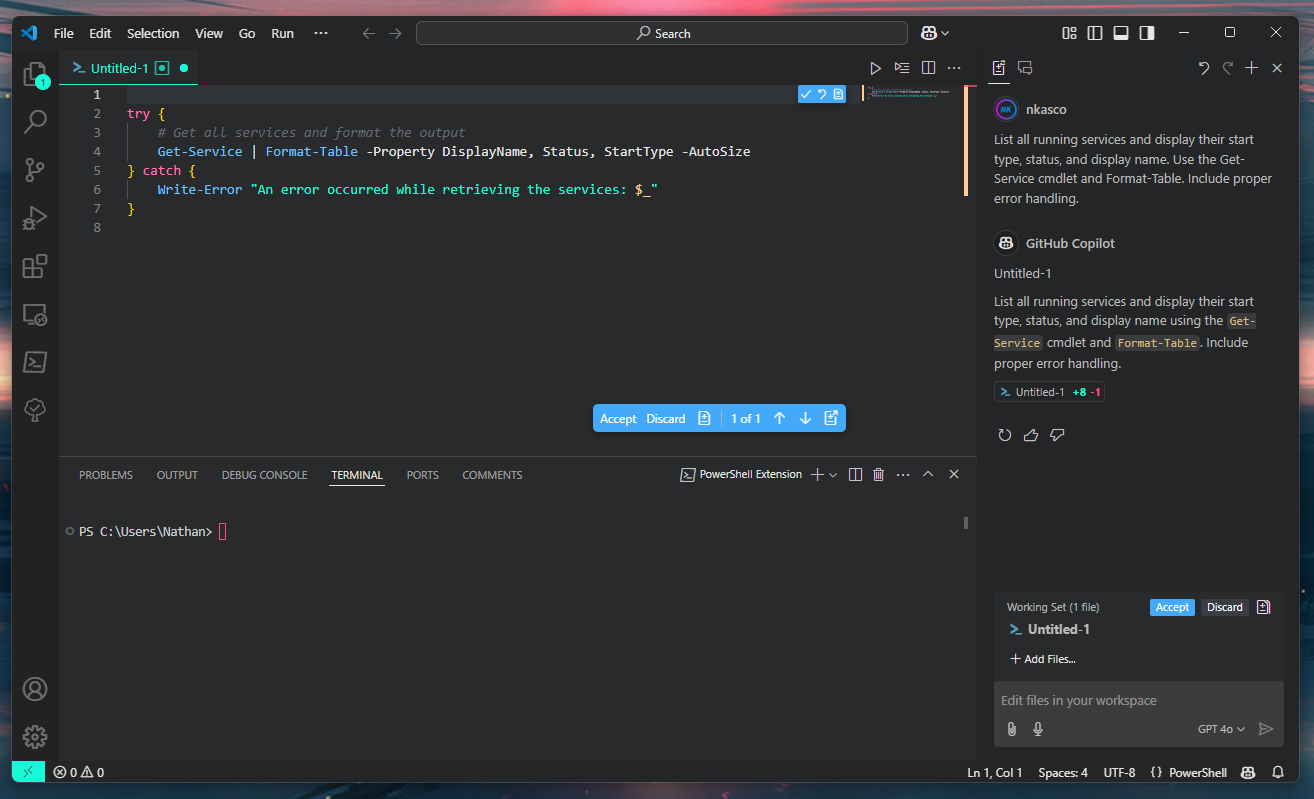
- Accept or Reject Changes
- For each file, you can accept, reject, or partially apply the suggested edits.
- This granular control ensures you only incorporate the changes you agree with.
- Iterate if Needed
- If Copilot’s first pass isn’t exactly what you had in mind, refine the
Also include the service’s DisplayName property in the output, and make sure to format columns to fit nicely.
- Copilot will propose a revised set of changes.
- If Copilot’s first pass isn’t exactly what you had in mind, refine the
By using Copilot Edits, you’re effectively taking a quick inline snippet and turning it into a more robust conversation with the AI—particularly useful when the logic spans multiple files or requires a series of iterative improvements.
Tips and Tricks for Maximizing Productivity
- Use Natural Language Comments: Clear, descriptive comments yield the best suggestions.
- Iterate with Feedback: Don’t be afraid to reject a suggestion and refine your prompt.
- Leverage Multi‑File Context: When working on interconnected scripts, add your relevant files to the working set so Copilot sees the bigger picture.
- Stay Updated: GitHub continues to roll out updates—such as support for multiple models from Anthropic, Google, and OpenAI—so keep an eye on the latest features.
Conclusion
Integrating GitHub Copilot into my PowerShell workflow has transformed how I develop scripts—reducing routine tasks and allowing me to focus on problem-solving. With features like Copilot Edits, the tool is evolving from a simple autocomplete assistant into a comprehensive AI‑pair programmer. I encourage you to try it out, experiment with your prompts, and see firsthand how it can enhance your daily work.
Happy scripting!
Learn More
If you’re interested in learning more about GitHub Copilot and its latest features, check out these official resources:
For updates, community discussions, and more insights, be sure to visit the official GitHub pages and join the conversation.
Leave a Reply